A Simple Serial (I2C/SPI) EEPROM Programmer 23 December 2014 on electronics, tools, tgl6502 This is a small ATtiny84 based device to program I2C and SPI EEPROM chips over a serial port. As usual all code and schematics are available in GitHub.
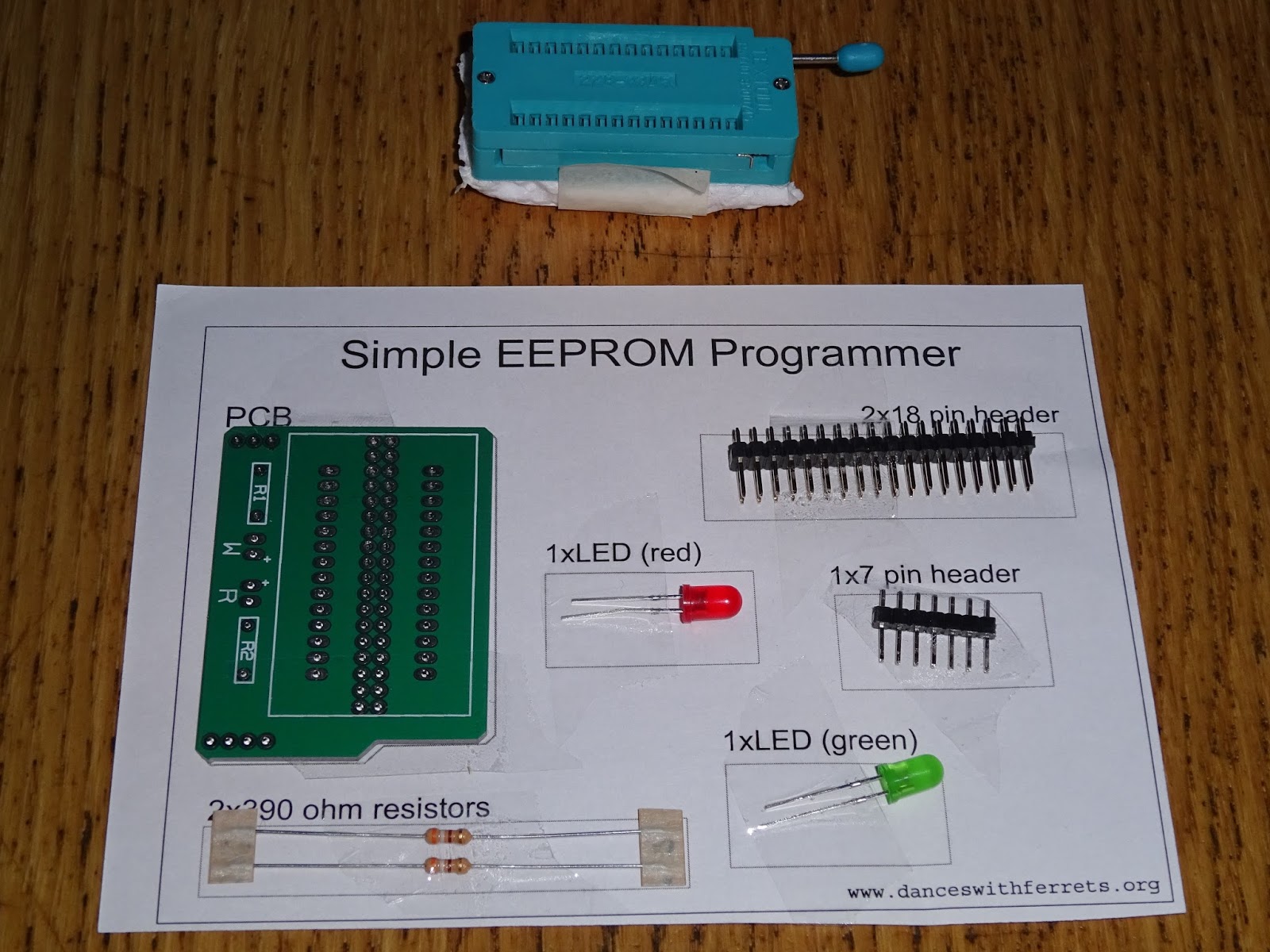
In this project we are building a JDM programmer that can handle PIC12, PIC16 and PIC18 family microcontrollers and some popular 24C family EEPROMs. The programmer also provides ICSP feature that allows In-Circuit Serial Programming. So if you desire, you will not have to carry your MCU each time when you reprogram it. EE-Prog is an USB 24 series EEPROM programmer. Its Hardware is based on AVR ATMega8 microcontroller. 24 series EEPROM programmer. All 24c EEPROM (24c01 - 24c1024. SIMPLE EPROM PROGRAMMER A simple EPROM programmer is described here. It connects to a PC's parallel port and can program EPROMs from 2716 to 27512. The design can be expanded to program.
2003-04-18
Here is a simple serial (I2C) EEPROM programmer for the PC parallel port. I built it to program 24XX devices, but it can, in principle at least, talk to any I2C device with a suitable adapter or fly-lead, it implements a complete I2C bus interface.
The original prototype and control software was developed against a 24LC16B device, but has since been extended and tested against the 24LCX range with X in { 08, 16, 21, 32, 64, 128, 256 }. It should work fine with other 24X I2C EEPROM devices, perhaps with slight changes to the addressing logic and sizing for larger devices like the 24LC512 (and 24LC1024 when it becomes available). Adding an extra device is generally just a matter of adding an extra struct near the top of 24xx.c and recompiling.
One exception might be the power supply. The 24LC16B and other similar chips pull just a little more than 3 mA when writing to power the internal programming voltage generator (less when reading, and virtually nothing in standby). The programmer is port powered but the parallel port outputs are specified to source only 3.5 mA. That is cutting it a bit fine, but in practice it has worked perfectly on several different PC parallel ports.
Power is sourced from the C0 bit, on some weird parallel ports this may be open collector rather than being capable of sourcing current. If yours is one of them, or you suffer other power problems, you can try pulling power from D2-D7 and C2-C3 with extra diodes, they are all pulled up by the software. If all else fails, supply an external 5 V supply. The diodes will protect the port if external power is supplied, especially if you intend to use the programmer as a generic I2C interface.
Note the switch/jumper in the diagram that allows selection of the state of the /WP signal on most devices. This was added after support for the 24LC21A was requested by Miika Ahdesmäki. The 24LC21A's /VCLK signal has the opposite sense to the usual /WP, as do several others designed for plug-n-play data service. The driver was also modified to pre-clock it as per the requirements in its datasheet to get it into the bi-directional operation mode.
A bi-directional parallel port is not required as the SDA and SCL lines are read through the Error and Paper-Out status bits (S3 and S4). The C1 bit drives a power indicating red LED (or a 'don't remove chip' warning if you prefer). The D0 and D1 lines drive the pull-down of SDA and SCL, they have a yellow and green LED to display their states. (The state of the pull-down drive, not SDA and SCL themselves! You may wish to add two more transistors to drive the LEDs from the bus state instead, making them more useful.)
You can download my Linux user space programmer drivers. They call ioperm() to establish access to the I/O space for the parallel port so they must be run as root, or setuid root. Currently the I/O base is hardcoded for the 1st parallel port at 0x378. The code is interactive, entering '?' at the prompt will give you a list of options:
Commands: q Quit ? This usage message d Dump EEPROM, as hex D <start> <len> Dump EEPROM, as hex f <value> Erase EEPROM, filling with value r <filename> Dump EEPROM into raw binary file w <filename> Program EEPROM from raw binary file t <type> Set EEPROM device type (as 24C<type>) p <delay> Set delay between I2C transitions (for slower devices)
It is fairly primitive right now, it only supports raw binary input and output files, but I intend to add support all the common hex-file formats. It already has the option to dump the data pretty-printed in hex for manual inspection, and can fill the device with a specified byte to clear it or test it for bit failures near its end of life.
It would not be very challenging to make this code portable to Win32 console, just replace the inb/outb() and ioperm() calls. All else should port directly. This is true for all remotely POSIX systems, as long as they have some facility to allow user space programs to invoke I/O instructions.
10 comments.
This is a small ATtiny84 based device to program I2C and SPI EEPROM chips over a serial port. As usual all code and schematics are available in GitHub.
Background
The TGL-6502 project uses an SPI EEPROM (the Microchip 25AA1024) to simulate the ROM exposed to the 6502 processor. To get the content into the ROM I added a simple serial protocol to the TGL-6502 firmware but as the firmware grew this functionality had to be dropped to free up some of the limited flash memory so I had to find an alternative method.
I considered using a generic tool such as the Bus Pirate to program the EEPROMS or even investing in a generic programmer (there are a wide range available on eBay that support various MCU chips as well as EEPROMs). In the end I decided to build my own - the EEPROM programming protocol is very straight forward and I would be needing it for future projects as well.
As well as supporting SPI devices I wanted to be able to program I2C EEPROMs as well (the Raspberry Pi HAT specification uses an I2C EEPROM to provide information about the expansion board) - at this stage the hardware for I2C support is in place but there is no firmware support for that protocol yet, I will add it as I need it.
Hardware Design
I am using an ATtiny84 in 14 pin DIP format as the main CPU for the project. This chip has enough IO lines to do everything needed, more than enough flash to allow for more complex firmware and is small enough to keep the board fairly compact. The circuit could easily be modified to use an ATmega though if that is what you have available.
The circuit is very simple, apart from the CPU the only other electrical components are three resistors and a diode. The first two resistors pull the I2C lines (SDA and SCL) high and the third pulls the ATtiny84 RESET line high. Rather than use a serial bootloader I added a 10 pin AVR ISP header on the board for programming the firmware, the diode is used to isolate the VCC lines from the ISP header and the FTDI connector.
The rest of the components are connectors, the 10 pin ISP header I mentioned, a 6 pin FTDI connector and an 18 pin ZIF (Zero Insertion Force) socket for mounting the target EEPROM in. Using the ZIF socket reduces the risk of damaging the pins on the EEPROM - I had an 18 pin socket in my parts collection already, you can swap it out for two 8 pin DIP sockets if you want.
The serial connection and power come from a 6 pin FTDI Friend connector. The ones I use are switchable between 3.3V and 5.0V so when programming 3.3V EEPROM chips I just ensure that I have the FTDI adapter switched to the correct voltage level.
Firmware Design
To make development a bit easier I am using an Arduino core for the ATtiny84 and the firmware is implemented as an Arduino sketch. There were a few hardware limitations of the ATtiny that needed to be worked around in software though.
The ATtiny doesn't have a UART so there is no hardware serial port support - the serial port needs to be implemented in software by driving the IO pins directly at the right time. I didn't have a lot of luck with the Arduino SoftwareSerial library, I could not get reliable serial communications working at any speed. I wound up migrating the serial implementation from my tinytemplate library for the ATtiny85 and using that instead which gives me reliable communications at 57600 baud.

The USI (Universal Serial Interface) module on the ATtiny is used to implement both I2C and SPI but you can only use one protocol at a time. This in itself is not a problem (you will only be programming an I2C or an SPI EEPROM, not both simultaneously) but some of the pins overlap (SCL and SCK for example) which would complicate the circuit and routing. Because SPI is a lot easier to simulate in software (using the shiftIn() and shiftOut() functions in the Arduino library) I reserve the USI module for I2C and selected the SPI interface pins based on how easy they were to route.
One problem I did have in this project is the timer interrupts - the Arduino library uses an interrupt triggered by TIMER0 for timing functions (delay(), millis() and the like). This interrupt seemed to be causing issues with the SPI communications so I disabled it in the init() function:
In this case I'm not using any of the timer functions so it doesn't effect the rest of the code.
The remainder of the firmware deals with memory buffer management and protocol handling. The current implementation takes up a little over 4K, around half of the available space, which leaves a lot of room for enhancements.
Control Protocol
The programmer is controled over a serial port (57600 8/N/1) using an ASCII ping/pong protocol. You send a command terminated by a line feed character and wait for a response terminated by a line feed character. The response must be received before the next command can be sent. There are 5 available commands, outlined below:
With the exception of RESET the first character of the response will indicate success ('+') or failure ('-') and there may be additional information between the result character and the end of the line. For the read command this is hex data, for other commands any additional characters can be treated as an informational message.
The details of each command are described below, you can use a serial terminal to talk directly to the programmer but don't include the '<' and '>' characters shown in the examples - they are used to indicate the direction of the data.
RESET
Best Eprom Programmer
This should be the first command sent to the device - it will set the device into an idle state and ensure the power to the EEPROM slots is turned off. Unlike the other commands this one does not respond with a +/- success or failure indication - instead it reports the programmer identification string and the firmware version.
After receiving the reset command (and responding with the identity string) the programmer will go into IDLE mode.
Eeprom Programmer Software
INIT
This command is used to tell the programmer the type and specifications of the EEPROM it is dealing with. For each EEPROM we need to know a number of parameters:
- The EEPROM protocol - SPI or I2C.
- The size of the EEPROM. I use the number of bits in the address to determine this.
- The size of the EEPROM write page. This is the smallest amount of memory that can be written at once, once again I use the number of bits to determine the size (eg: a 32 byte page is 6 bits, 256 bytes is 8).
- The number of bytes of address to send on the SPI bus.
This information is encoded in a 16 bit integer as shown below.
The 16 bit value is sent as hex with the INIT command and the programmer will respond with success if the configuration is acceptable.
The following table shows the ID codes for some of the Microchip EEPROMs I have been using:
Part | Size | Page Size | Address Bytes | ID Code |
---|---|---|---|---|
25AA1024 | 1Mbit (128K x 8) | 256 bytes | 24 bit | 0x7830 |
25LC1024 | 1Mbit (128K x 8) | 256 bytes | 24 bit | 0x7830 |
25AA640 | 64Kbit (8K x 8) | 32 bytes | 16 bit | 0x4620 |
READ
Use this command to read data from the EEPROM. The command character is followed by a 3 byte address in hexadecimal and a successful response is the 3 byte address, a sequence of data bytes and a 2 byte checksum.
The checksum is simply a sum of all bytes in the response (excluding the checksum itself) and the lowest 16 bits of the value is used as the checksum. The code to do this looks like the following:
WRITE
This command is used to begin or continue a write sequence. Once the first write command has been accepted you can continue writing to sequential addresses or send a DONE command (described below) to finish the sequence and return to READY mode.
The format of the WRITE command is similar to the response from the READ command - a 3 byte address, a sequence of data bytes and a 2 byte checksum. The checksum is calculated in the same way as for READ - simply sum the byte values in the line into a 16 bit integer ignoring overflow.
Note that the write command will buffer data into RAM until it has a full page to write to the EEPROM - you must use the DONE command to terminate a write sequence to ensure all data has actually been written. If the buffer only contains a partial page the rest of the contents will be filled with whatever is already in the EEPROM allowing you to do partial page writes to patch the data in the EEPROM rather than doing a complete rewrite.
DONE
All write sequences must be terminated with this command. If there is a partial page still in the RAM buffer it will be filled with the current contents of the EEPROM and written. The command then returns to READY mode allowing you to issue READ commands or start another WRITE sequence.
The EEPROG Utility
The repository includes a simple Windows GUI utility to control the programmer in the software/eeprog directory.
You can compile this utility with the Visual Studio Community Edition - it's a simple Windows Forms application written in C#. The utility doesn't make use of all the functionality of the programmer - it simply allows you to burn an arbitrary binary file to the target EEPROM or read the contents of the EEPROM to a binary file. In most cases this will be all that you need.
Next Steps
The tool currently provides all the functionality I need to work on the TGL-6502 but there are obviously a few enhancements that can be made. So far I have only tested the device with Microchip SPI EEPROM devices which all have the same command set - supporting other manufacturers devices may require providing additional information in the EEPROM identity word to select alternative command sets.
Eeprom Programmer Download
Support for I2C devices is built in to the hardware but not yet implemented in the firmware. I intend to use the Arduino Wire library to communicate with these chips.
The programming utility for Windows could be extended to support Intel HEX format files as well as raw binary which would be useful for dealing with output from linkers. The ability to set the start address for programming would also come in handy rather than having to prepare a complete EEPROM image for every burn. More importantly a command line utility that could be incorporated into make files is a must.
Spi Eeprom Programmer
All of these enhancements are relatively simple to implement and I will modify the code to support them as the need arises. If you make the changes yourself (or add interesting new functionality) please send me a pull request and I'll add them to the main repository.
